General Explanation Of The Code
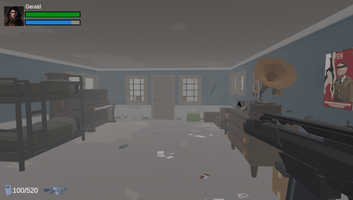
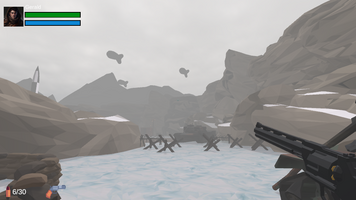
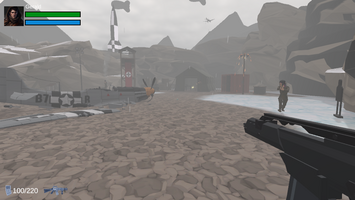
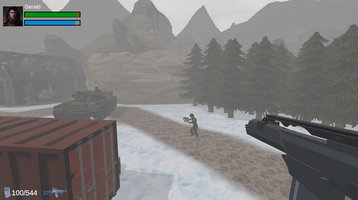
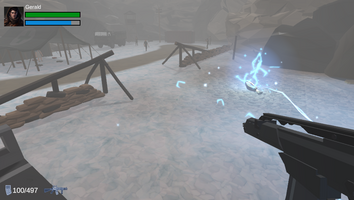
Enemy AI
This project contains an artificial intelligence script for an enemy in a video game. The enemy is capable of patrolling, chasing, and attacking the player according to certain conditions.
main features
Player Perception: The enemy is able to sense the player's presence within a certain range and react accordingly.
Patrolling: When the enemy cannot see the player, he patrols between a set of predefined waypoints. If there are no established waypoints, the enemy simply stays at their current position.
Pursuit: If the enemy spots the player, it will start chasing the player while staying at a certain distance.
Attack: When the enemy is close enough to the player, they will perform attacks against him.
Reaction to Player Attacks: If the player attacks the enemy, the enemy will change his behavior to focus on the player.
How to use this script
Add this script to an enemy object in your Unity project. Make sure the enemy has a properly configured NavMeshAgent and Animator component. Also, assign a reference to the player object in the scene.
Set public script variables such as patrol speed, pursuit speed, sight and attack range, and parry range to adjust enemy behavior to your needs.
If you want the enemy to patrol between waypoints, add transforms to the waypoints list in the Unity Inspector.
Additional notes
The script also includes audio events that can be used to play sounds when the enemy starts and stops chasing the player. You can assign these events to the corresponding audio clips in the Unity Inspector.
Enemy behavior can be modified and extended as needed to suit the needs of your project.
FireGun: Grenade firing and launching system for Unity
This C# script is designed to be used in a Unity project, and is responsible for implementing a firing and grenade throwing system for a character in a 3D game. The script allows you to switch between different weapons, shoot, throw grenades, reload ammunition, and manage visual and sound effects.
main features
• Damage control for different weapons.
• Switch between different types of weapons
• Pistol and rifle shooting
• Gun muzzle flash effects
• Blood and impact effects on walls
• Launch and explosion of grenades
• Configuration of maximum distance, speed and effects of the bullets.
• Blood, wall impact and cannon flash effects.
• Control of the type of weapon and 3D objects of the weapons.
• Rate and accuracy of weapons.
• Sounds and effects for weapons and grenades.
• Current, maximum and total ammunition control.
• Weapon change and UI update.
Use
Add this script to the object that controls the character in Unity.
Assign property values in the Unity Inspector, such as effect prefabs, sounds, weapon and bullet sprites, etc.
Make sure that items tagged "Enemy" have a health script (like EnemyHealth in this example) so that the damage system works properly.
Add the FireGun script to an object in the scene, such as the player character.
Set up script variables in the Unity Inspector to customize weapons, effects, and sounds.
Add 3D models of weapons and the effects of blood, wall impact and muzzle flash.
Add the UIManager component and configure the UI to display weapons and ammo.
main methods
• Start(): Initializes the AudioSource component and the current weapons ammunition.
• Update(): Controls weapon switching, firing, grenade throwing, and reloading on each frame.
• ChangeWeapon(): Changes the current weapon and updates the UI.
• Shoot(float accuracy): Fires the weapon and deals damage to enemies if it hits them.
• ThrowGrenade(): Throws a grenade and deals damage to enemies within the blast radius.
• ExplodeGrenade(GameObject grenade, float delay): Controls the explosion of the grenade after a while.
• Reload(): Weapon reload method.
• Damage Weapons: Controls the damage of weapons.
• Config Bullet: Controls the maximum distance, the bullet, the point of fire and the speed of the bullet.
• FX: Controls the effects of blood, wall impact and cannon flash.
• Type Gun: Control the type of weapon and the 3D objects of the weapons.
• Accuracy Rate: Controls the cadence and precision.
• Sound: Controls the sounds of weapons and the AudioSource component.
• Img Sprite: Controls the images of weapons and bullets in the UI.
• Grenade: Controls the grenade and launch point, damage, damage radius, and grenade sounds and effects.
• Ammo: Controls the current and maximum ammunition of the weapons.
• Sound FX: Controls the firing and reloading sounds.
limitations
• The script does not include reloading or weapon switching animations.
• There is no weapon upgrade system.
PlayerHealth - Character health and shield system in Unity
This script allows you to manage a character's health and shield in a Unity game. Health and shield are visually represented by health and shield bars, and are automatically updated based on damage taken.
Characteristics
• Management of the character's health and shield
• Images of life and shield bars
• Method of taking damage
• Verification of character death
Use
Add the PlayerHealth script to an object in the scene, such as the player character.
Set up script variables in the Unity Inspector to customize max health, max shield, and health and shield bar images.
Make sure other scripts that deal damage to the character call the TakeDamage() method.
variables
The PlayerHealth script uses several variables to control the character's health and shield, as well as the images of the health and shield bars:
• maxHealth: The character's maximum health.
• maxShield: The character's maximum shield.
• lifeBar: The image of the life bar.
• shieldBar: The image of the shield bar.
• health: The character's current health.
• shield: The character's current shield.
• shieldDamageReduction: The damage reduction applied by the shield.
• isDead: A boolean indicating whether the character is dead or not.
methods
The PlayerHealth script includes several methods to manage the character's health and shield:
• Awake(): Initializes the static Obj instance of the script.
• Start(): Initializes the character's health and shield.
• Update(): Updates the character's health and shield, as well as the images of the health and shield bars, and checks if the character has died.
• TakeDamage(float damage): Applies damage to the character's health and shield.
• OnDestroy(): Cleans the static Obj instance of the script.
limitations
• The script does not include any animation or death effects.
• There is no max health or max shield enhancement system.
Live to die
Live to die
Status | Prototype |
Author | fhposso |
Genre | Shooter |
Tags | First-Person |
Leave a comment
Log in with itch.io to leave a comment.